This post will help you to go throw the GDB debugger quickly. You are to start a C project from scratch under Linux (I’m using Ubuntu 15.10 distribution).
If you can find this post, I presume that you have the basic knowledge on what GDB is.
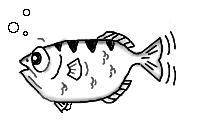
Create Project
Create Workspace
To get start, first let’s create a workspace. Press Ctrl + Alt + t to open a Terminal.1
2
3
4
5
6
7
8mkdir workspace # create a directory under current directory
cd workspace # enter the directory
mkdir learnGDB # create a directory for GDB learning
cd learnGDB # enter the above directory
touch main.cc # create a main file
touch foo.cc # create a c file
touch foo.h # create a header file
Edit Code
The following code is used to demonstrate the usage of the GDB. From main program call a function, the function returns a const integer. If you are a Vim newbee, google how to use vim to edit the C file.
Follows the previous progress, in the terminal:1
vim main.cc
Write the following code.1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
int main(int argc, char* argv[]){
int z = 2;
int* x = &z;
int* y = &z;
z = foo(x, y);
printf("z = %d\n", z);
return 0;
}
Quit vim (Esc, :wq, Enter). Edit header file.1
vim foo.h
Write program1
2
3
4
5
6
int foo(int* x, int* y);
Quit vim (Esc, :wq, Enter).1
vim foo.cc
Edit the c function code.1
2
3
4
5
6
7
8
int foo(int* x, int* y){
*x = 0;
*y = 1;
return *x;
}
Quit vim (Esc, :wq, Enter).
Compile Project
Under the work directory.1
2
3
4
5
6
7
8
9
10
11
12
13gcc -I./ -g foo.cc main.cc -o learnGDB -std=c99
:<<'BLOCK
........ This is comments ............
.... gcc is the compiler
.... -I./ means to search the current directory for files.
.... -g means keep debug information, this is the prerequest of using GDB
.... -o learnGDB means output binary is learnGDB
.... -std=c99 means using c99 standard.
........ This is the end of comments ............
BLOCK'
./learnGDB
z = 1
If you can have “z = 1” on terminal, then it works.
Using GDB
Under the same directory.1
2
3
4
5
6
7
8
9
10
11
12gdb learnGDB
b main
r
n
l
b foo
c
n
n
...
n
exit
gdb learnGDB means use gdb to start debug.
b main means add break point at the main function.
r means start to run
l means list the program
b foo means add break point at the foo function.
c means continue until meets the next break point.
n means next step.
exit means step out of gdb.
Other useful commands
gdb -tui learnGDB: use UI to do the debugging.
step (s): step into a function.
until (u): step out of the for loop.
until + line number: run to the specified line.
finish: run until the current function returns.
call: call a visable function in the current program. e.g. call foo(3, 4).
break n: add a break point at the nth line.
print a: print variable a’s value.
watch: Run the program if the being watched variable’s value changed. e.g. watch a.
layout asm: display disassembly code.
clear: clear break points.
…
More commands can be found gdb commands cheat sheet.
Listen to the music and take a break. [Somewhere] of July. Cheers~
License
The content of this blog itself is licensed under the Creative Commons Attribution 4.0 International License.
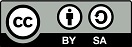
The containing source code (if applicable) and the source code used to format and display that content is licensed under the Apache License 2.0.
Copyright [2016] [yeephycho]
Licensed under the Apache License, Version 2.0 (the “License”);
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
Apache License 2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an “AS IS” BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
express or implied. See the License for the specific language
governing permissions and limitations under the License.
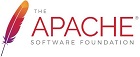